728x90
Linear Regression (선형회귀) : 데이터들을 가장 잘 표현하는 직선의 방정식을 찾는 것
Data 를 대표하는 hypothesis를 직선의 방정식으로 설정
y1 = W1x1 W2x2 + B1
y2 = W3x1 W4x2 + B2
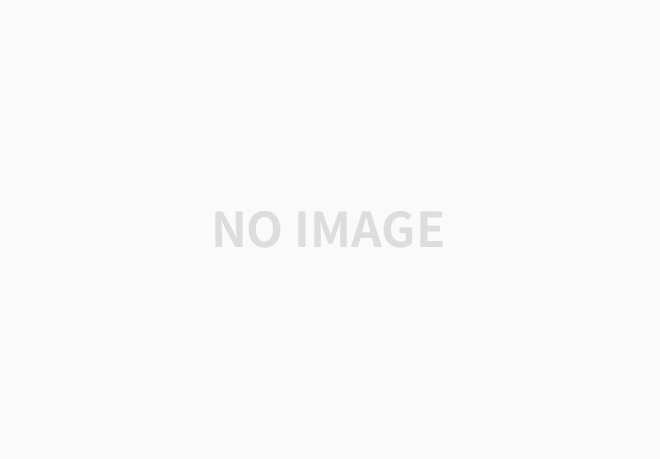
Step1) Hypothesis
Hypothesis : Linear Equation(Multi-Variable)
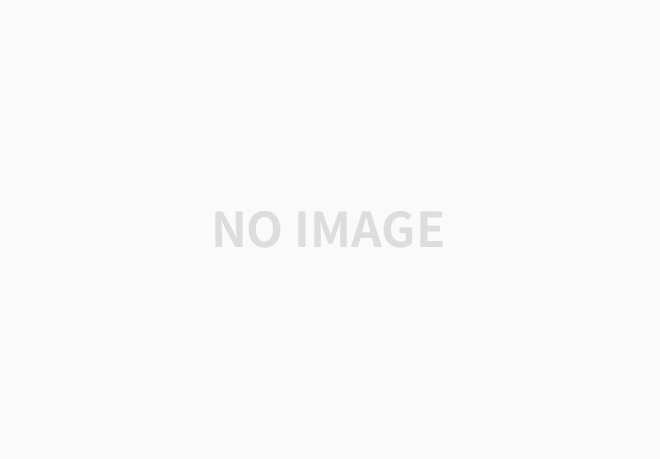
Step2) Cost function
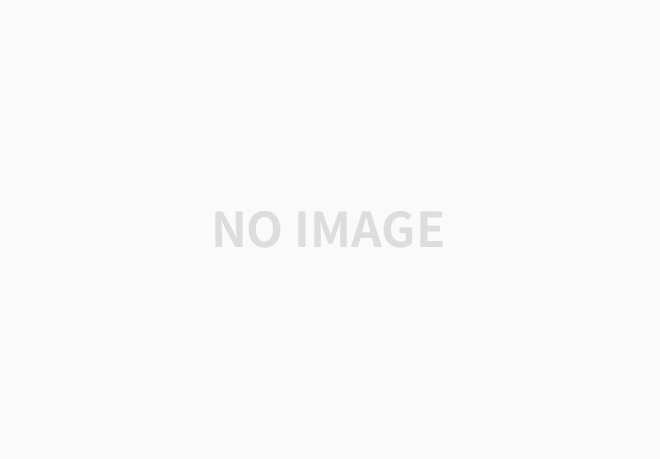
Step3) Training - gradient descent method
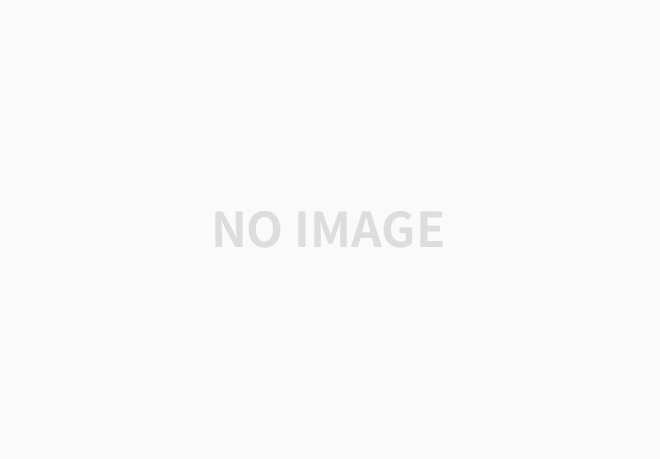
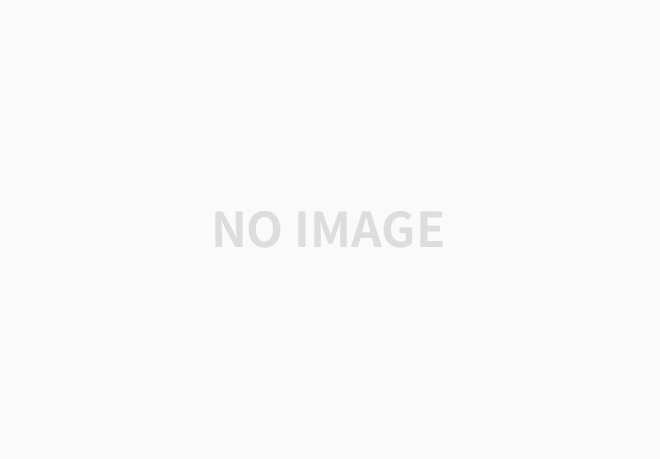
Tensorflow
w/ min-max scaling
#load module
import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
#input & label (age/BMI and high/low blood pressure)
x_input = tf.constant([[25,22],[25,26],[25,30],[35,22],[35,26],[35,30],[45,22],[45,26],[45,30],
[55,22],[55,26],[55,30],[65,22],[65,26],[65,30],[73,22],[73,26],[73,30]], dtype= tf.float32)
labels = tf.constant([[118,72],[125,80],[130,80],[118,76],[126,75],[123,79],[120,80],[124,85],[130,83],
[122,78],[125,80],[130,81],[127,75],[130,79],[130,81],[125.5,80],[130,80],[138,82]], dtype= tf.float32)
W = tf.Variable(tf.random.normal((2, 2)), dtype=tf.float32)
B = tf.Variable(tf.random.normal((2,)), dtype=tf.float32)
#min-max scaling
x_input_org = x_input
xmin, xmax = np.min(x_input, axis = 0), np.max(x_input, axis = 0)
x_input = (x_input - xmin)/(xmax-xmin)
#Function Generation
def Hypothesis(x) :
return tf.matmul(x,W) + B
def Cost() :
return tf.reduce_mean(tf.square(Hypothesis(x_input)-labels))
def Predict(x):
return Hypothesis((x-xmin)/(xmax-xmin))
#Parameter
epochs = 10000
lr = 0.01
opt = tf.keras.optimizers.SGD(learning_rate=lr)
training_idx = np.arange(0, epochs+1, 1)
cost_graph = np.zeros(epochs+1)
#Training
for cnt in range(0, epochs+1):
cost_graph[cnt] = Cost()
if cnt % (epochs//10) == 0:
print("[{:>6}] cost={:>6.4}, W = [[{:>7.4} {:>7.4}] [{:>7.4} {:>7.4}]], B = [{:>7.4}{:>7.4}]".
format(cnt, cost_graph[cnt], W[0,0], W[0,1], W[1,0],W[1,1], B[0],B[1]))
opt.minimize(Cost,[W, B])
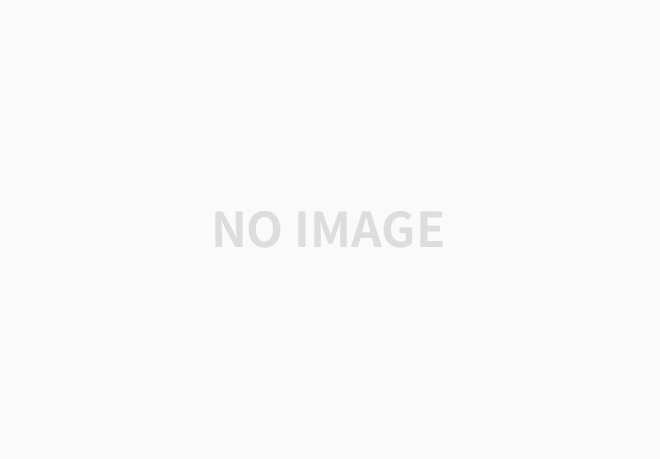
#Validation Test
H_x = Hypothesis(x_input)
for x,h,l in zip(x_input_org, H_x, labels):
print("Age:{}, BMI:{}=>BP:{:>7.4},{:>7.4} [label => {}]".format(x[0],x[1],h[0], h[1],l))
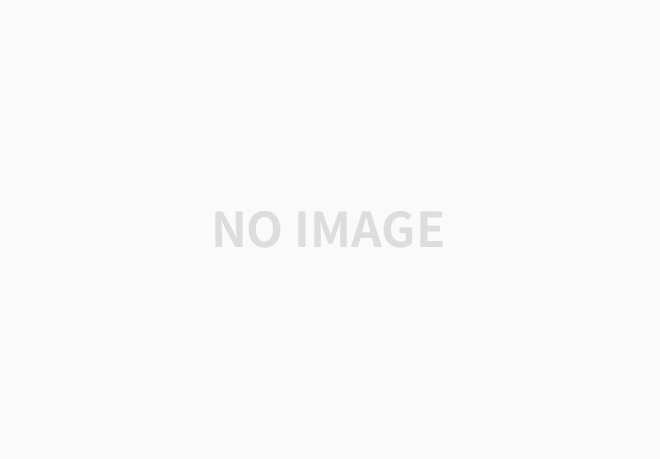
#Prediction
age = 29.0
BMI = 25.0
test = tf.constant([[age,BMI]], dtype = tf.float32)
print("Age - {}, BMI - {} : {}mmHg".format(age, BMI, Predict(test)))
Age - 29.0, BMI - 25.0 : [[121.81335 77.56746]]mmHg
#Cost function graph
plt.title("'Cost / Epochs' Graph")
plt.xlabel("Epochs")
plt.ylabel("Cost")
plt.plot(training_idx, cost_graph)
plt.xlim(0, epochs)
plt.grid(True)
plt.semilogy()
plt.show()
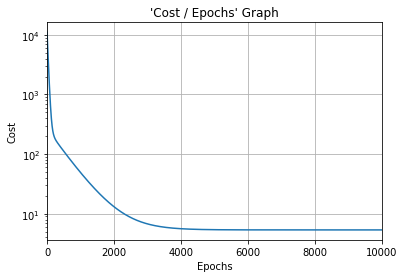
728x90
'Machine Learning' 카테고리의 다른 글
Supervised Learning - Logistic Regression (multi-variable 2) (0) | 2023.08.06 |
---|---|
Supervised Learning - Logistic Regression (multi-variable 1) (0) | 2023.08.05 |
Supervised Learning - Linear Regression (multi-variable 1) (0) | 2023.08.05 |
Supervised Learning - Linear Regression (single-variable) (0) | 2023.08.05 |
Machine Learning 개요 (0) | 2023.08.05 |